<%@ Page Language="C#" %>
<%@ Import Namespace="System.Web.Configuration" %>
<script runat="server">
void GridView1_PreRender(object sender, EventArgs e)
{
if (GridView1.SelectedRow != null)
{
Table table = GridView1.SelectedRow.Parent as Table;
if (table != null)
CreateRow(table, GridView1.SelectedIndex);
}
}
void CreateRow(Table table, int index)
{
GridViewRow row = new GridViewRow(-1, -1, DataControlRowType.DataRow, DataControlRowState.Normal);
row.Cells.Add(CreateColumn());
table.Rows.AddAt(index + 2, row);
}
private TableCell CreateColumn()
{
TableCell cell = new TableCell();
cell.ColumnSpan = GridView1.Columns.Count;
cell.Width = Unit.Percentage(100);
DataSourceControl ds = CreateDataSourceControl();
cell.Controls.Add(ds);
cell.Controls.Add(CreateDetailsView(ds));
return cell;
}
private static DetailsView CreateDetailsView(DataSourceControl ds)
{
DetailsView dv = new DetailsView();
dv.AutoGenerateRows = true;
dv.DataSourceID = ds.ID;
return dv;
}
private DataSourceControl CreateDataSourceControl()
{
SqlDataSource ds = new SqlDataSource();
ds.ConnectionString = WebConfigurationManager.ConnectionStrings["MyConnectionString"].ConnectionString;
ds.SelectCommand = "SELECT * FROM [Users] WHERE [UserID] = @UserID";
ds.ID = "SqlDataSource2";
Parameter cp = new Parameter("UserID", TypeCode.String, GridView1.SelectedValue.ToString());
ds.SelectParameters.Add(cp);
return ds;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml" >
<head id="Head1" runat="server">
<title>Untitled Page</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" Runat="server" DataSourceID="SqlDataSource1" DataKeyNames="UserID"
AutoGenerateColumns="False" OnPreRender="GridView1_PreRender" EnableViewState="False">
<Columns>
<asp:CommandField ShowSelectButton="True">
<asp:BoundField ReadOnly="True" HeaderText="UserID" DataField="UserID" SortExpression="UserID">
<asp:BoundField HeaderText="UserName" DataField="UserName" SortExpression="UserName"></asp:BoundField>
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" Runat="server" SelectCommand="SELECT [UserID], [UserName] FROM [Users]"
ConnectionString="<%$ ConnectionStrings:MyConnectionString %>">
</asp:SqlDataSource>
</div>
</form>
</body>
</html>
Tech Tidbits - Ruby, Ruby On Rails, Merb, .Net, Javascript, jQuery, Ajax, CSS...and other random bits and pieces.
Wednesday, August 27, 2008
Dynamic Details Row in GridView
Saturday, August 23, 2008
Amazon S3 with Ruby aws-s3 gem
I stated using Amazon S3 to store and backup the music I've written and recorded. I'm using the Ruby aws-s3 gem. Note that I check for some basic audio file types so I don't have to manually type the bucket or mime-type.
I can call the script a couple ways:
# with defaults based on extension
$ ruby aws-s3.rb mysong.mp3
# or explicitly (file, bucket, mime-type)
$ ruby aws-s3.rb mysong.mp3 doug_mp3 'audio/mp3'
aws-s3.rb
---------
#!/usr/bin/env ruby
require 'rubygems'
require 'aws/s3'
# File to upload
local_file = ARGV[0]
# bucket & mime-type
if local_file =~ /mp3$/
bucket = 'doug_mp3'
mime_type = 'audio/mpeg'
elsif local_file =~ /wav$/
bucket = 'doug_wav'
mime_type = 'audio/x-wav'
elsif local_file =~ /aif[c|f]?$/
bucket = 'doug_aiff'
mime_type = 'audio/x-aiff'
else
bucket = ARGV[1]
mime_type = ARGV[2] || "application/octet-stream"
end
if bucket.nil?
puts "need a bucket"
exit
end
puts "file: #{local_file}"
puts "bucket: #{bucket}"
puts "mime_type: #{mime_type}"
AWS::S3::Base.establish_connection!(
:access_key_id => 'REPLACE_ME',
:secret_access_key => 'REPLACE_ME'
)
base_name = File.basename(local_file)
puts "Uploading #{local_file} as '#{base_name}' to '#{bucket}'"
AWS::S3::S3Object.store(
base_name,
File.open(local_file),
bucket,
:content_type => mime_type
)
puts "Uploaded!"
I can call the script a couple ways:
# with defaults based on extension
$ ruby aws-s3.rb mysong.mp3
# or explicitly (file, bucket, mime-type)
$ ruby aws-s3.rb mysong.mp3 doug_mp3 'audio/mp3'
Add and remove a remote source from gem 'remote sources''
I was attempting to add a gem and ran into an error I hadn't seen before:
So I tried to update gems:
Next I looked the gems environment:
Noticing the issues with Merb (which I recently installed), I removed http://merbivore.com from the list of remote sources:
I went ahead and added github while I was at it:
Checked my gems environment:
Everything looked good, so I tried to install aws-s3 gem again:
Et VoilĂ ! C'est si bon...
Macintosh:aws-s3 dsparlingimbp$ sudo gem i aws-s3
WARNING: RubyGems 1.2+ index not found for:
http://merbivore.com
RubyGems will revert to legacy indexes degrading performance.
Updating metadata for 63 gems from http://gems.rubyforge.org/
...............................................................
complete
ERROR: could not find gem aws-s3 locally or in a repository
So I tried to update gems:
Macintosh:aws-s3 dsparlingimbp$ sudo gem update --system
Updating RubyGems
WARNING: RubyGems 1.2+ index not found for:
http://merbivore.com
RubyGems will revert to legacy indexes degrading performance.
Updating metadata for 63 gems from http://gems.rubyforge.org/
...............................................................
complete
ERROR: While executing gem ... (Gem::RemoteFetcher::FetchError)
bad response Not Found 404 (http://merbivore.com/Marshal.4.8)
Next I looked the gems environment:
Macintosh:rubygems-1.2.0 dsparlingimbp$ gem env
RubyGems Environment:
- RUBYGEMS VERSION: 1.2.0
- RUBY VERSION: 1.8.6 (2007-03-13 patchlevel 0) [i686-darwin8.8.5]
- INSTALLATION DIRECTORY: /usr/local/lib/ruby/gems/1.8
- RUBY EXECUTABLE: /usr/local/bin/ruby
- EXECUTABLE DIRECTORY: /usr/local/bin
- RUBYGEMS PLATFORMS:
- ruby
- x86-darwin-8
- GEM PATHS:
- /usr/local/lib/ruby/gems/1.8
- GEM CONFIGURATION:
- :update_sources => true
- :verbose => true
- :benchmark => false
- :backtrace => false
- :bulk_threshold => 1000
- :sources => ["http://gems.rubyforge.org", "http://merbivore.com"]
- REMOTE SOURCES:
- http://gems.rubyforge.org
- http://merbivore.com
Noticing the issues with Merb (which I recently installed), I removed http://merbivore.com from the list of remote sources:
sudo gem sources -r http://merbivore.com
I went ahead and added github while I was at it:
sudo gem sources -a http://gems.github.com
Checked my gems environment:
Macintosh:rubygems-1.2.0 dsparlingimbp$ gem env
RubyGems Environment:
- RUBYGEMS VERSION: 1.2.0
- RUBY VERSION: 1.8.6 (2007-03-13 patchlevel 0) [i686-darwin8.8.5]
- INSTALLATION DIRECTORY: /usr/local/lib/ruby/gems/1.8
- RUBY EXECUTABLE: /usr/local/bin/ruby
- EXECUTABLE DIRECTORY: /usr/local/bin
- RUBYGEMS PLATFORMS:
- ruby
- x86-darwin-8
- GEM PATHS:
- /usr/local/lib/ruby/gems/1.8
- GEM CONFIGURATION:
- :update_sources => true
- :verbose => true
- :benchmark => false
- :backtrace => false
- :bulk_threshold => 1000
- :sources => ["http://gems.rubyforge.org", "http://gems.github.com"]
- REMOTE SOURCES:
- http://gems.rubyforge.org
- http://gems.github.com
Everything looked good, so I tried to install aws-s3 gem again:
Macintosh:rubygems-1.2.0 dsparlingimbp$ sudo gem install aws-s3
Successfully installed aws-s3-0.5.1
1 gem installed
Installing ri documentation for aws-s3-0.5.1...
Installing RDoc documentation for aws-s3-0.5.1...
Et VoilĂ ! C'est si bon...
Subscribe to:
Posts (Atom)
About Me
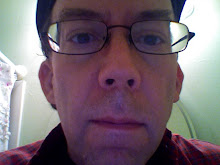
- Doug Sparling
- Developer (Ruby on Rails, iOS), musician/composer, Buddhist, HSP, Vegan, Aspie.
Labels
- .NET (8)
- accordion (1)
- ActiveRecord (1)
- ajax (2)
- APT (1)
- apt-get (1)
- ASP (1)
- ASP.NET .NET (5)
- Audio (1)
- aws (1)
- Bash (1)
- bdd (1)
- C# (1)
- cache_fu (1)
- caching (1)
- DocBook (1)
- DOM (1)
- Eclipse (1)
- Excel (1)
- Firefox (1)
- gem (5)
- Gems (5)
- git (1)
- GridView (2)
- Hibernate (1)
- iBATIS (1)
- Java (9)
- javascript (9)
- javascript css (1)
- jQuery (4)
- jsspec (1)
- mdb2 (2)
- Merb (2)
- mongrel (2)
- mp3 (2)
- Music (2)
- MySQL (2)
- nginx (1)
- openssl (1)
- osx (3)
- pdocast (1)
- pear (2)
- Perl (7)
- php (5)
- plugin (2)
- podcast (1)
- prototype (1)
- REST (1)
- RMagick MacOSX (1)
- rspec (1)
- ruby (14)
- ruby on rails (21)
- RubyGems Merb (1)
- s3 (1)
- screencasts (1)
- scriptaculous (1)
- scriptrunner ruby rails erlang (1)
- Sortable (1)
- subversion (1)
- testing (1)
- testing erlang tsung (1)
- tomcat (1)
- ubuntu (2)
- WebSphere (1)
- will_paginate (1)